Vue.js Conditions & Loops
Conditions and Loops are used in all programming languages to provide repetitive control structures. They can repeat one or more various steps depending on the conditions. Same we can use in the case of Vue.js.
v-if Directive Example
Index.html file:
Index.js file:
Note: Here, we have used a simple CSS file to make the output more attractive. This CSS file will be the same for all the following examples.
Index.css file:
Output:
This is visible to you
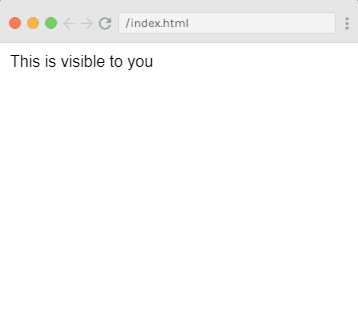
In the above example, if you enter app3.seen = false, you will see that the message disappears.
Example2:
Index.html file:
Index.js file:
Output:
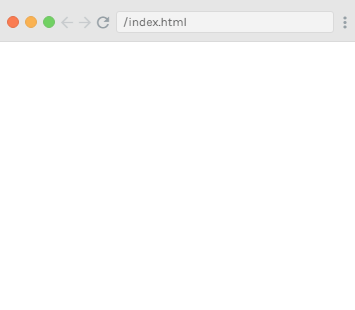
In the above example, you can see that you can bind data to not only text and attributes, but also the structure of the DOM. Vue also provides a powerful transition effect system that can automatically apply transition effects when the elements are inserted, updated, or removed by Vue.
v- else-if Directive Example
We can use the v- else-if directive to provide an else functionality in the above example. By using this directive, if the condition is not satisfied or not true then it will return the else statement that you set for the program. Let's see an example.
Index.html file:
Index.js file:
Output:
You can't see me
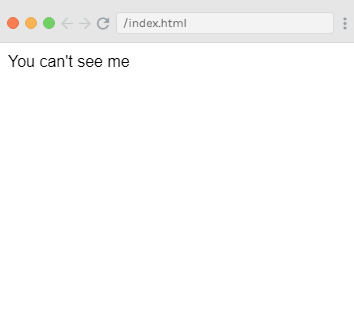
In the above example, you can see that the output is shown as "You can't see me" which is set as v-else-if statement. There are also some other directives in Vue.js that can be used for their own special functionalities.
v- for Directive Example
The v-for directive is used to display a list of items using the data from an Array. See the following example.
Index.html file:
Index.js file:
Output:
- HTML Tutorial
- CSS Tutorial
- JavaScript Tutorial
- AngularJS Tutorial
- js Tutorial
Output:
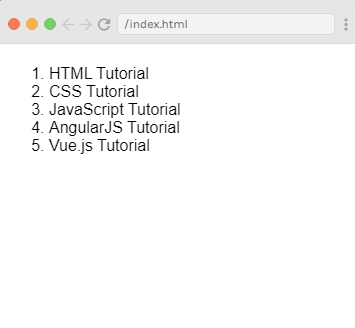
Handling User Input
The v-on directive facilitates users to interact with your Vue.js app. It is used to attach event listeners that invoke methods on Vue instances. Let's see an example of v-on directive.
v-on Directive Example
Index.html file:
Index.js file:
Output:
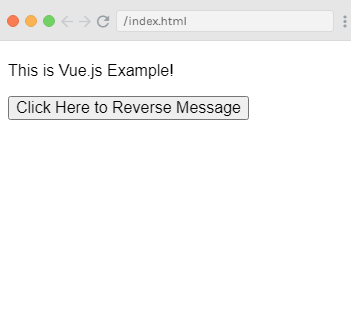
After clicking on the "Click Here to Reverse Message" button, the above string will be reversed.
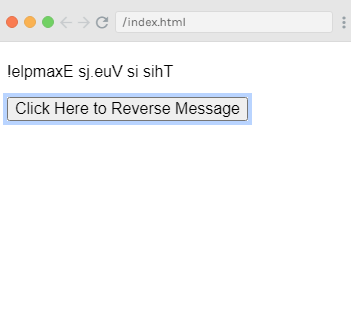
In the above example, the state of app is updated without touching the DOM. All DOM manipulations are handled by Vue itself.
v-model Directive Example
The v-model directive is used to make two-way data binding between form input and app state. See the following example:
Index.html file:
Index.js file:
Output:
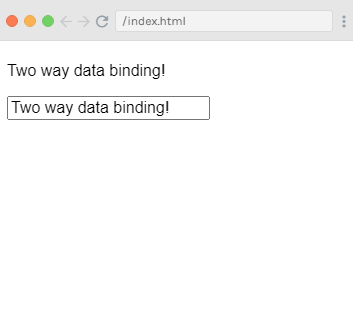
You will see the automatic synchronization of data in the above example. When you change the data in one component, the change will be reflected in both components. See the below example:
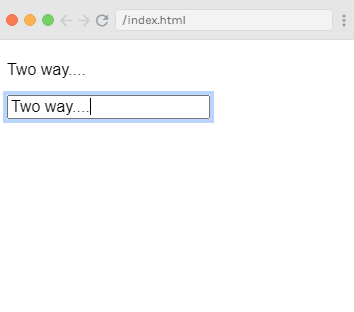
Composing with Components
The component system is used when we want to build large-scale applications composed of small, self-contained, and often reusable components. A large application interface can be abstracted into a tree of components:
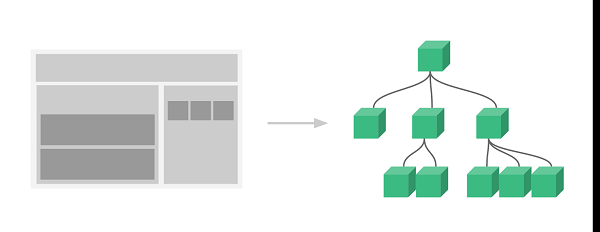
Here, we use the v-bind directive to pass values in repeated component. See the following example:
Index.html file:
Index.js file:
Output:
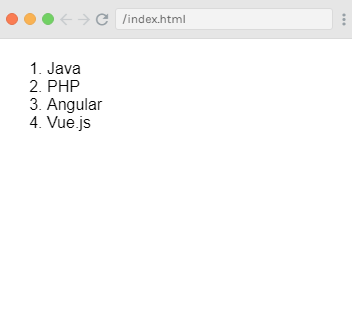
0 comments:
Post a Comment
Thanks