
10 November, 2023
How to pass multiple values as sql array for a parameter? (Or how can I cirumvent limit of parameters of prepared statement?)
Programing Coderfunda
November 10, 2023
No comments
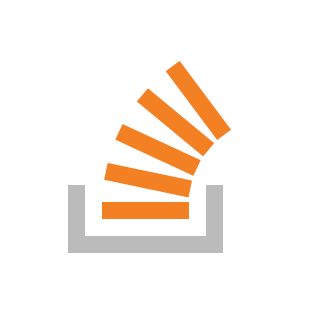
How to get the cell's output data from a azure databricks notebook to a file
Programing Coderfunda
November 10, 2023
No comments
Getting an image from the database using path images on the client side
Programing Coderfunda
November 10, 2023
No comments
09 November, 2023
The Ultimate "git nah" Alias
Programing Coderfunda
November 09, 2023
No comments
Laravel Nova gets a fresh new website
Programing Coderfunda
November 09, 2023
No comments
How to prevent GitHub Actions from being run based on name of the branch merging into other branch
Programing Coderfunda
November 09, 2023
No comments
How to prevent dialog from disappearing? Reactjs
Programing Coderfunda
November 09, 2023
No comments
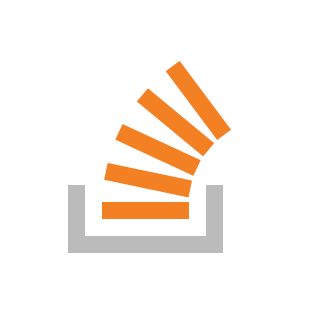
Using route group in NextJS 13 stops tailwind from working
Programing Coderfunda
November 09, 2023
No comments
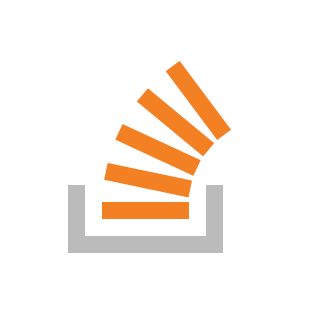
08 November, 2023
Laravel Pail - The easiest way to tail your log files
Programing Coderfunda
November 08, 2023
No comments
Laravel 10.31 Released
Programing Coderfunda
November 08, 2023
No comments
SwiftUI / Xcode: ForEach closed range vs half open range - error with Text function. Why the difference?
Programing Coderfunda
November 08, 2023
No comments
I do not understand why PostgreSQL indicates that a procedure does not exist
Programing Coderfunda
November 08, 2023
No comments
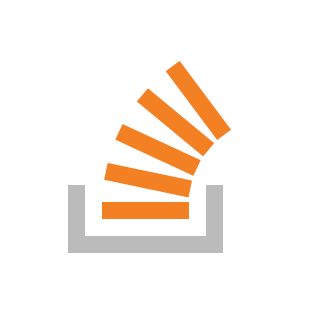
I can't get new data after apolo fetchMore
Programing Coderfunda
November 08, 2023
No comments
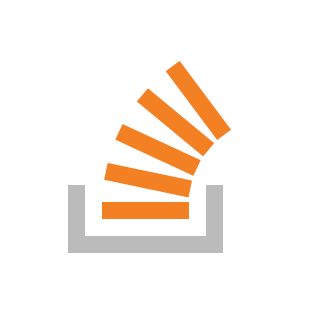
07 November, 2023
forEach is not a function (for translation tool) [closed]
Programing Coderfunda
November 07, 2023
No comments
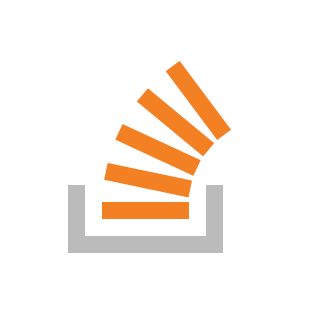
Marklogic SVC-FILRD error even when file is present on the filesystem
Programing Coderfunda
November 07, 2023
No comments
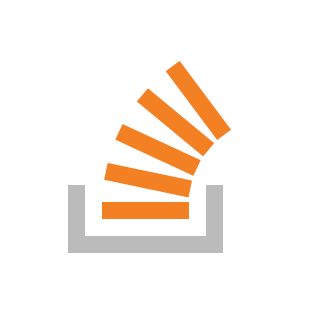
Map two different entities to the same table?
Programing Coderfunda
November 07, 2023
No comments
The user is following himself
Programing Coderfunda
November 07, 2023
No comments
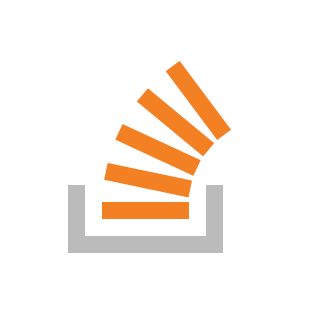
OnCollisionEnter does not work correctly at high speeds?
Programing Coderfunda
November 07, 2023
No comments
06 November, 2023
ABP Problem with loading @abp/ng.identity module
Programing Coderfunda
November 06, 2023
No comments
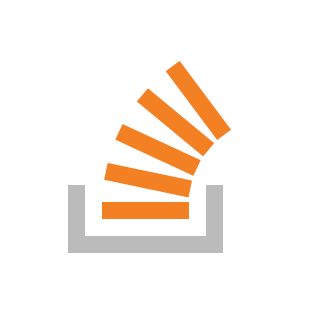
Recreating bar chart with R and ggplot2
Programing Coderfunda
November 06, 2023
No comments
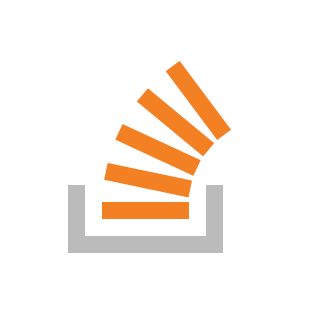
Apache Pulsar implementation in a distributed system
Programing Coderfunda
November 06, 2023
No comments
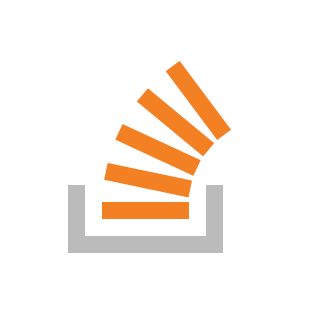
SQL or NoSQL batabase for online strategy
Programing Coderfunda
November 06, 2023
No comments
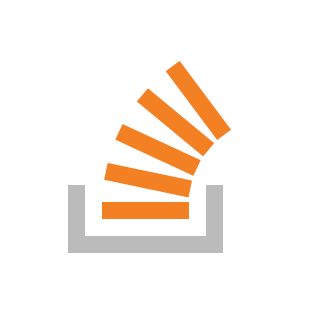
Could not access file "$libdir/age": No such file or directory in Windows
Programing Coderfunda
November 06, 2023
No comments
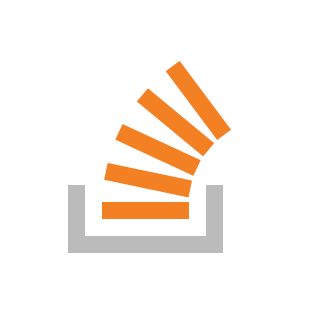
05 November, 2023
Should the card board or just the card be statefull?
Programing Coderfunda
November 05, 2023
No comments
NSwag openapi2cscontroller: handle controller return type for errors
Programing Coderfunda
November 05, 2023
No comments
create a directory name from a string in python
Programing Coderfunda
November 05, 2023
No comments
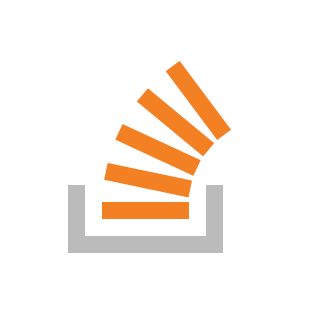
Disable audit object on oracle 11
Programing Coderfunda
November 05, 2023
No comments
How to create a new Bitbucket pull request via API?
Programing Coderfunda
November 05, 2023
No comments
04 November, 2023
How to unload assemblies without using AppDomain.Unload?
Programing Coderfunda
November 04, 2023
No comments
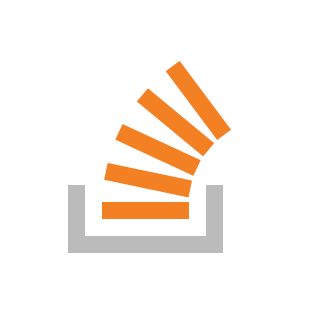