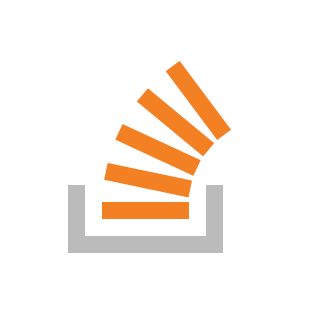
How to type two variables that are correlated in TypeScript?
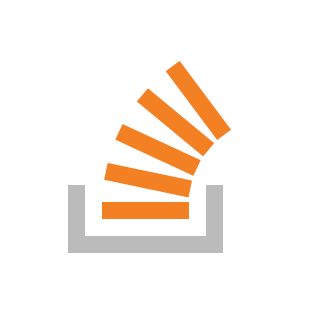
Programing Coderfunda
March 06, 2024
No comments
Programing Coderfunda
March 06, 2024
No comments
Programing Coderfunda
March 06, 2024
No comments
Programing Coderfunda
March 06, 2024
No comments
Programing Coderfunda
March 06, 2024
No comments
Programing Coderfunda
March 05, 2024
No comments
Programing Coderfunda
March 05, 2024
No comments
Programing Coderfunda
March 05, 2024
No comments
Programing Coderfunda
March 05, 2024
No comments
Programing Coderfunda
March 05, 2024
No comments
Programing Coderfunda
March 04, 2024
No comments
Programing Coderfunda
March 04, 2024
No comments
Programing Coderfunda
March 04, 2024
No comments
Programing Coderfunda
March 04, 2024
No comments
Programing Coderfunda
March 04, 2024
No comments
Programing Coderfunda
March 03, 2024
No comments
Programing Coderfunda
March 03, 2024
No comments
Programing Coderfunda
March 03, 2024
No comments
Programing Coderfunda
March 03, 2024
No comments
Programing Coderfunda
March 03, 2024
No comments
Programing Coderfunda
March 02, 2024
No comments
Programing Coderfunda
March 02, 2024
No comments
Programing Coderfunda
March 02, 2024
No comments
Programing Coderfunda
March 02, 2024
No comments
Programing Coderfunda
March 02, 2024
No comments
Programing Coderfunda
March 01, 2024
No comments
Programing Coderfunda
March 01, 2024
No comments
Programing Coderfunda
March 01, 2024
No comments
Programing Coderfunda
March 01, 2024
No comments
Programing Coderfunda
March 01, 2024
No comments